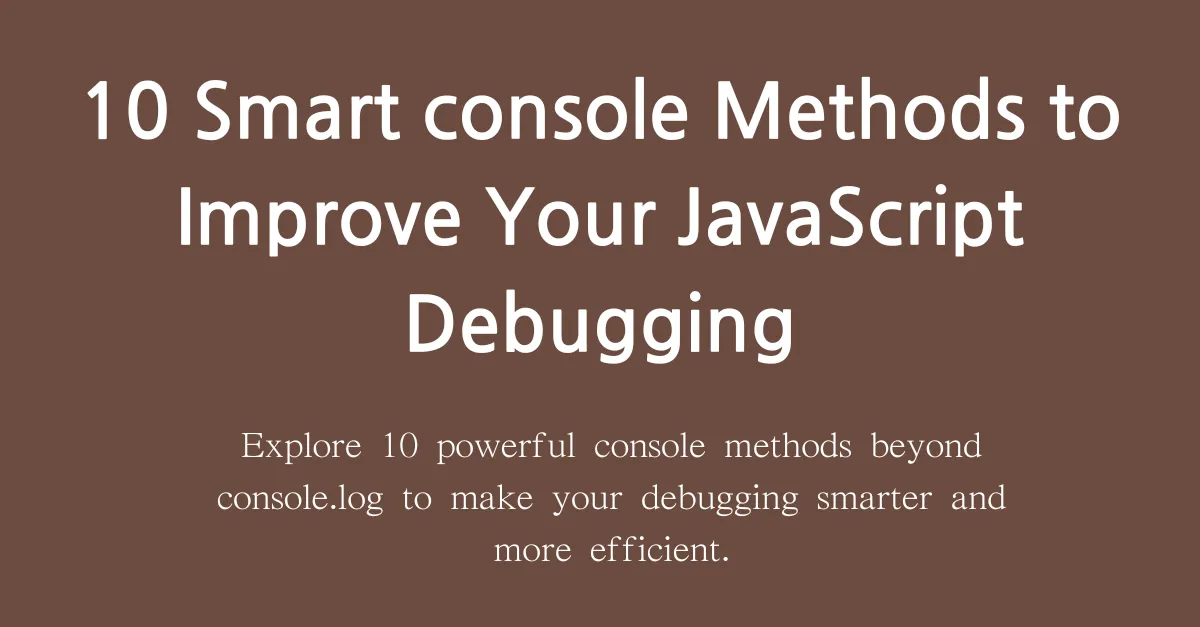
10 Smart console Methods to Improve Your JavaScript Debugging
Explore 10 powerful console methods beyond console.log to make your debugging smarter and more efficient.
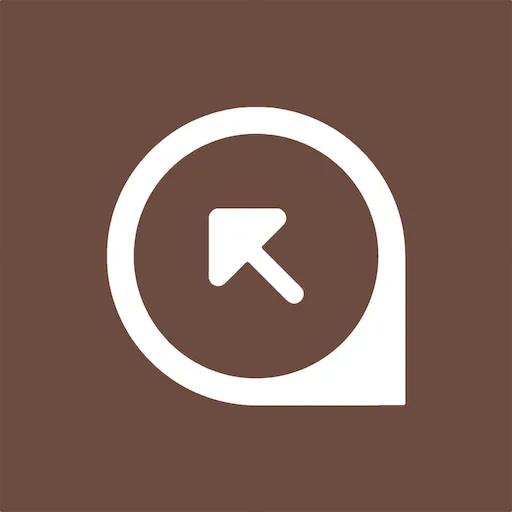
10 Smart console Methods to Improve Your JavaScript Debugging #
Let’s go beyond console.log()
and explore more powerful tools for smarter debugging.
Most developers rely on console.log()
during development, and it’s often the first step when checking whether values are correct or code is functioning properly. However, the console
object provides many more useful methods that can make debugging more efficient and your code more organized.
Here are 10 powerful console
methods every JavaScript developer should know:
1. console.error()
#
Highlights error messages in red. Use it when you want to make critical issues or exceptions stand out.
console.error("An error occurred!");
2. console.warn()
#
Displays warning messages in yellow. Ideal for non-critical issues users should be aware of.
console.warn("This is a warning!");
3. console.info()
#
Outputs informational messages. Often similar in appearance to console.log()
, depending on the browser.
console.info("This is an informational message.");
4. console.table()
#
Displays arrays or objects in a nice tabular format. Especially helpful for inspecting JSON data.
const users = [
{ name: "Chulsoo", age: 25 },
{ name: "Younghee", age: 30 }
];
console.table(users);
5. console.dir()
#
Outputs an interactive list of an object’s properties. Useful for inspecting HTML elements or deep object structures.
const obj = document.body;
console.dir(obj);
6. console.group()
/ console.groupEnd()
#
Groups related logs together in a collapsible format, making nested logs easier to follow.
console.group("User Info");
console.log("Name: Chulsoo");
console.log("Age: 25");
console.groupEnd();
7. console.time()
/ console.timeEnd()
#
Measures how long a block of code takes to run — useful for performance monitoring.
console.time("Execution Time");
for (let i = 0; i < 1000000; i++) {}
console.timeEnd("Execution Time");
8. console.assert()
#
Logs a message only if the condition is false.
const value = 1;
console.assert(value === 2, "Value is not equal to 2!");
9. console.clear()
#
Clears the console output.
console.clear();
10. console.count()
/ console.countReset()
#
Counts the number of times a specific label has been called.
function greet(name) {
console.count("Greet Count");
console.log(`Hello, ${name}!`);
}
greet("Chulsoo");
greet("Younghee");
console.countReset("Greet Count");
greet("Minsu");
Bonus: console.trace()
#
Prints a stack trace to show the code path taken to reach the current point.
console.trace("Trace call");
Try It Yourself – Sample HTML #
Here’s a simple HTML file to test all of the above:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>Console Methods Demo</title>
</head>
<body>
<h1>Open Developer Tools (F12) to See the Console Output</h1>
<script>
console.log("✅ General log");
console.error("❌ An error occurred!");
console.warn("⚠️ This is a warning!");
console.info("ℹ️ Info log");
const users = [
{ name: "Chulsoo", age: 25 },
{ name: "Younghee", age: 30 }
];
console.table(users);
const obj = document.body;
console.dir(obj);
console.group("👤 User Info");
console.log("Name: Chulsoo");
console.log("Age: 25");
console.groupEnd();
console.time("⏱ Execution Time");
for (let i = 0; i < 1000000; i++) {}
console.timeEnd("⏱ Execution Time");
const value = 1;
console.assert(value === 2, "❗ value is not 2!");
console.count("Greet Count");
console.count("Greet Count");
console.countReset("Greet Count");
console.count("Greet Count");
console.trace("📍 Trace point");
console.groupCollapsed("📦 Collapsed group");
console.log("This group is collapsed by default");
console.groupEnd();
setTimeout(() => {
console.clear();
console.log("🧼 Console has been cleared!");
}, 10000);
</script>
</body>
</html>
Why You Should Clean Up console
Logs in Production #
Leaving too many console logs in production code can lead to:
- Performance issues due to excessive rendering in developer tools.
- Security concerns from leaked data like user info or internal logic.
- Messy code that’s harder to maintain and review.
- Larger bundle sizes due to unnecessary logs.
Best Practices to Remove Console Logs Before Deployment #
✅ Use Babel Plugin #
Install a plugin to automatically remove console logs during production builds:
npm install --save-dev babel-plugin-transform-remove-console
Then configure it in .babelrc
:
{
"env": {
"production": {
"plugins": ["transform-remove-console"]
}
}
}
✅ Use ESLint #
Warn or error when console.log()
is used:
// .eslintrc.js
module.exports = {
rules: {
"no-console": "warn" // or "error"
}
};
✅ Create a Custom Logger #
Use a wrapper to conditionally log messages based on the environment:
// logger.js
export const logger = {
log: (...args) => {
if (process.env.NODE_ENV !== "production") {
console.log(...args);
}
}
};
import { logger } from './logger';
logger.log("Debugging message");
✅ Static Analysis Tools #
Use tools like eslint
, sonarqube
, husky
, prettier
, or even grep:
grep -rnw './src' -e 'console.log'
Conclusion #
The console
object is more than just log()
. Mastering its other methods can help you debug faster, cleaner, and more effectively. Just don’t forget to remove or manage those logs before going live!